In this android example we learn how to access android system app / call
activity on a button click event. here on click button access default
SMS , Dialer , Contact and web browser or selected by you.
Step 1: Write code into activity_main.xml
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity" >
<Button
android:id="@+id/butdialer"
style="?android:attr/buttonStyleSmall"
android:layout_width="match_parent"
android:layout_height="50dp"
android:layout_marginTop="28dp"
android:text="Dialer" />
<Button
android:id="@+id/butsearch"
style="?android:attr/buttonStyleSmall"
android:layout_width="match_parent"
android:layout_height="50dp"
android:layout_marginTop="36dp"
android:text="Search" />
<Button
android:id="@+id/butsms"
style="?android:attr/buttonStyleSmall"
android:layout_width="match_parent"
android:layout_height="50dp"
android:layout_marginTop="48dp"
android:text="SMS" />
<Button
android:id="@+id/butcontact"
style="?android:attr/buttonStyleSmall"
android:layout_width="match_parent"
android:layout_height="50dp"
android:layout_marginTop="37dp"
android:text="Contact" />
</LinearLayout>
Step 2: Write code into MainActivity.java
package dev.androidapplink.system
import android.os.Bundle;
import android.app.Activity;
import android.content.Intent;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//define controls
//define controls
Button mySmsBtn = (Button) findViewById(R.id.butsms);
Button myDialerBtn = (Button) findViewById(R.id.butdialer);
Button myContactBtn = (Button) findViewById(R.id.butcontact);
Button mySearchBtn = (Button) findViewById(R.id.butsearch);
//implement button click event
myContactBtn.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
Intent myIntent = new Intent();
myIntent.setAction(Intent.ACTION_VIEW);
myIntent.setData(android.provider.Contacts.People.CONTENT_URI);
startActivity(myIntent);
}
});
mySmsBtn.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
// TODO Auto-generated method stub
Intent openmsg = new Intent(Intent.ACTION_MAIN);
openmsg.addCategory(Intent.CATEGORY_DEFAULT);
openmsg.setType("vnd.android-dir/mms-sms");
startActivity(openmsg);
}
});
mySearchBtn.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
Intent myIntent = new Intent();
myIntent.setAction(Intent.ACTION_SEARCH);
startActivity(myIntent);
}
});
myDialerBtn.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
Intent myIntent = new Intent();
myIntent.setAction(Intent.ACTION_DIAL);
startActivity(myIntent);
}
});
}
}
NOTE: no need special permission in AndroidManifest.xml
Step 3: Now Run Your Project:
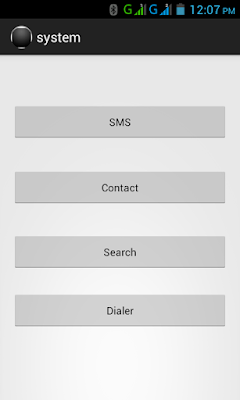
No comments :
Post a Comment